Enhancing Accessibility in React Native
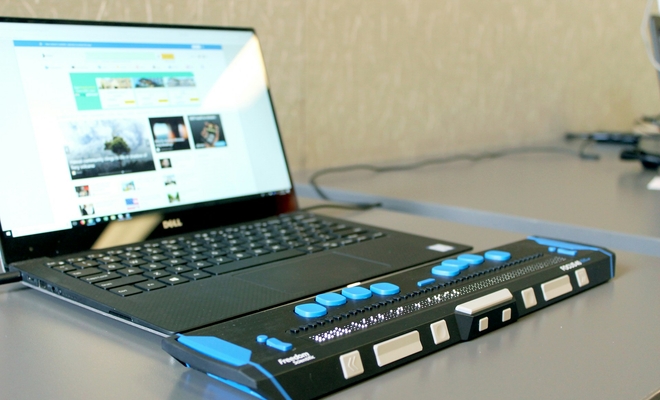
Introduction
Ensuring accessibility in React Native is crucial for making your apps usable by the widest possible audience, including people with disabilities. By leveraging React Native's built-in accessibility properties, developers can make their mobile applications more accessible to users with visual, auditory, and motor impairments. Let's explore how accessibility can be handled in React Native:
Accessible Properties
React Native provides several accessibility properties to enhance your app's usability:
-
accessible
: A Boolean prop that makes a component an accessibility element. When set totrue
, it combines its children into a single selectable component. -
accessibilityLabel
: Defines a label that screen readers announce to describe the element to visually impaired users. -
accessibilityHint
: Offers a brief description of the outcome of interacting with an element, providing additional context. -
accessibilityRole
: Defines the role of the component for screen readers, like "button", "link", "header", etc. -
accessibilityState
: Communicates component states, such as disabled or selected, to assistive technologies. -
accessibilityValue
: Provides numerical values for elements like sliders to assistive technologies. -
accessibilityActions
: Lists actions available to users beyond standard gestures. -
onAccessibilityAction
: Handles user-activated custom actions defined byaccessibilityActions
.
Implementing Accessibility
Here's an example integrating these properties into a React Native app:
import React from 'react';
import { View, Text, TouchableOpacity } from 'react-native';
const App = () => {
return (
<View>
<TouchableOpacity
accessible={true}
accessibilityLabel="Tap me"
accessibilityHint="Navigates to the next page"
accessibilityRole="button"
onPress={() => console.log('Button tapped')}
>
<Text>Next Page</Text>
</TouchableOpacity>
</View>
);
};
export default App;
Testing for Accessibility
- Manual Testing: Test with screen readers like TalkBack (Android) and VoiceOver (iOS).
- Automated Testing: Tools like axe and React Native Testing Library automate some accessibility checks.
Best Practices
- Contrast and Font Sizes: Ensure text is readable and allow for text scaling.
- Touchable Areas: Keep interactive elements at least 44x44 pixels for easy tapping.
- Descriptive Labels and Hints: Provide clear labels and hints for interactive elements.
- Avoid Color Only Indications: Do not rely solely on color to convey information.
By applying these practices, developers can make their React Native apps more accessible and provide a better experience for a wider audience.