Babel: Modern JavaScript Compatibility Made Easy
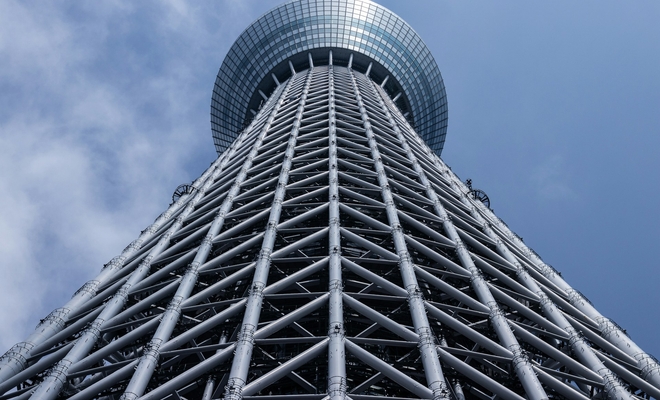
Babel: Modern JavaScript Compatibility Made Easy
Babel is a popular open-source JavaScript compiler that enables developers to write code using the latest JavaScript syntax and features, even if those features are not yet supported by all browsers or JavaScript environments. It compiles the code into backward-compatible JavaScript, allowing developers to adopt new features without worrying about browser compatibility.
Key Features of Babel
-
Transpilation: Babel converts JavaScript code written in newer versions of ECMAScript (like ES6/ES2015 and beyond) into a version that is compatible with older environments (e.g., ES5).
-
Plugins: Babel's functionality is extendable through plugins, which can be used to transform syntax, polyfill missing features, or optimize code.
-
Presets: Presets are collections of Babel plugins that work together to transform code in a specific way, making it easier to apply transformations for common scenarios.
-
Polyfills: Through plugins or libraries like
core-js
, Babel can add polyfills to inject missing functionalities into the environment. -
Modular: Babel's modular design allows developers to enable only the plugins and presets they need, resulting in more efficient code transformations.
Common Use Cases
-
Modern JavaScript Features: Babel is commonly used to transform modern JavaScript features like
async/await
, destructuring, and arrow functions into older syntax that is supported by a wider range of browsers. -
TypeScript: Babel can be configured to transpile TypeScript code to JavaScript, making it a versatile tool for projects using TypeScript.
-
React JSX: Babel can transform JSX syntax into regular JavaScript using plugins like
@babel/preset-react
. -
Polyfilling: With plugins like
@babel/preset-env
, Babel can include necessary polyfills for features not natively supported in the target environment.
How Babel Works
-
Parsing: Babel parses the JavaScript code into an Abstract Syntax Tree (AST), a structured representation of the code.
-
Transformation: Babel applies a series of plugins and presets to the AST, transforming it according to the specified configurations.
-
Generation: Babel generates new JavaScript code from the transformed AST.
Example of a Babel Configuration
A typical Babel configuration file (.babelrc
or babel.config.json
) includes:
-
Presets: A collection of plugins that are often used together for specific purposes.
{ "presets": ["@babel/preset-env", "@babel/preset-react"] }
-
Plugins: Individual transformations applied to the code.
{ "plugins": ["@babel/plugin-transform-runtime"] }
Popular Presets and Plugins
- @babel/preset-env: Automatically includes the necessary transformations for targeting specific JavaScript environments, based on the specified browsers.
- @babel/preset-react: Includes transformations for handling React's JSX syntax.
- @babel/plugin-transform-runtime: Reuses Babel's helper code to reduce code duplication and file size.
- @babel/plugin-proposal-class-properties: Enables support for class properties syntax.
Benefits of Using Babel
- Cross-Browser Compatibility: Allows developers to use modern JavaScript features without worrying about browser support.
- Improved Development Experience: Developers can use the latest JavaScript features, which often provide a more concise and expressive syntax.
- Ecosystem: Babel integrates well with other tools like webpack, allowing for seamless transformation in development workflows.
- Community Support: With a large and active community, Babel continuously evolves and supports the latest JavaScript features.
Conclusion
Babel has become an essential tool in modern JavaScript development, allowing developers to take advantage of the latest language features while maintaining compatibility with older environments. By using Babel, developers can write future-proof code that continues to run smoothly on a wide range of platforms.