Implementing Background Fetch in React Native
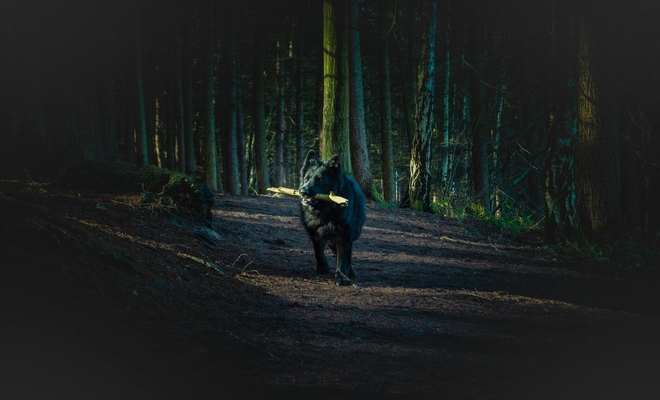
Implementing Background Fetch in React Native
React Native background fetch is a technique that allows your app to run background processes, primarily for updating content even when the app is not active or even closed. This feature is very useful for applications that need to update their data periodically (e.g., fetching new emails, updating weather information, synchronizing local data with a server, etc.).
How Background Fetch Works in React Native:
-
Periodic Execution: Background fetch mechanisms typically wake up the app at system-determined intervals and allow the app to run some background tasks. This wake-up frequency is controlled by the mobile operating system and is based on factors like device usage patterns and battery efficiency considerations.
-
Limited Execution Time: When a background fetch event occurs, the app is given a limited amount of time (usually around 30 seconds) to run its tasks and return. The tasks must be concise and efficient to fit within this time frame.
-
Task Completion Signal: The app needs to explicitly signal to the operating system when its background tasks have completed. This allows the OS to put the app back into a suspended state to conserve resources.
Implementation in React Native:
React Native does not natively support background fetch in its core API, so you typically would use a third-party library like react-native-background-fetch
by Transistor Software. Here’s a general approach to using this library:
Installation:
You would start by installing the library using npm or yarn:
npm install react-native-background-fetch
or
yarn add react-native-background-fetch
Basic Setup and Usage:
In your React Native application, you set up the background fetch like this:
import BackgroundFetch from "react-native-background-fetch";
class App extends Component {
componentDidMount() {
// Configure it.
BackgroundFetch.configure({
minimumFetchInterval: 15, // <-- minutes (15 is minimum allowed)
stopOnTerminate: false, // <-- Android-only,
startOnBoot: true, // <-- Android-only
}, async (taskId) => {
console.log("[js] Received background-fetch event: ", taskId);
// Perform your task here.
// IMPORTANT: You must signal to the OS that your task is complete.
BackgroundFetch.finish(taskId);
}, (error) => {
console.log("[js] RNBackgroundFetch failed to start");
});
// Optional: Check if the device supports background fetch
BackgroundFetch.status((status) => {
switch(status) {
case BackgroundFetch.STATUS_RESTRICTED:
console.log("Background fetch is disabled");
break;
case BackgroundFetch.STATUS_DENIED:
console.log("Background fetch is explicitly denied by the user for this app");
break;
case BackgroundFetch.STATUS_AVAILABLE:
console.log("Background fetch is available and enabled");
break;
}
});
}
}
Considerations:
- Platform Differences: Background fetch behaviors can differ significantly between iOS and Android. For example, Android apps can have more control over fetch intervals and can even run immediately on system startup, whereas iOS is more restrictive and uses machine-learning algorithms to determine the optimal times to initiate background fetches based on user habits.
- Battery and Data Usage: Excessive use of background fetch can lead to increased battery drain and data usage, which might frustrate users. It’s important to optimize the frequency and payload size of these background operations.
Using such libraries and adhering to platform-specific guidelines helps in implementing effective and efficient background fetch capabilities in React Native applications, keeping the app's data fresh while minimizing impact on device resources.