Understanding the Importance of Keys in React Native
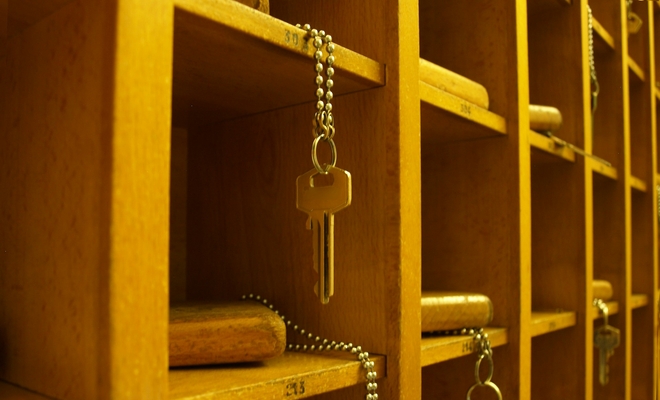
Understanding the Importance of Keys in React Native
In React Native, as in React, the key
prop plays a crucial role in managing the performance of lists and other collections of components. It is used to give elements a stable identity across re-renders, helping React optimize the rendering process by reusing existing elements in the DOM or, in the case of React Native, the native view hierarchy. Here’s how keys impact performance and functionality in React Native:
Purpose of Keys
- Identity: Keys help React identify which items in a list have changed, were added, or were removed. This information is crucial for efficiently updating the UI.
- Reconciliation: During the reconciliation process, React uses keys to match children in the old and new component trees. By providing a unique and consistent key for each component, React can minimize the amount of work needed to update the UI.
Best Practices for Using Keys
- Uniqueness: Each key must be unique among its siblings. However, they don't need to be globally unique in the entire component tree.
- Stability: Keys should be stable, not based on random values or indices that might change as items are added or removed from the list.
- Predictability: Prefer predictable keys that can be derived from the data itself, like an
id
property from an object that doesn’t change.
Common Usage Scenarios
- Lists: When rendering lists of components in React Native, such as using
FlatList
,SectionList
, or mapping over an array of data with.map()
, you should provide akey
prop to each list item.
Example
Here's a basic example of using keys in a React Native component that renders a list of items:
import React from 'react';
import { View, Text } from 'react-native';
const MyList = ({ items }) => {
return (
<View>
{items.map(item => (
<Text key={item.id}>
{item.text}
</Text>
))}
</View>
);
};
export default MyList;
In this example:
- Each
Text
component is assigned akey
that is theid
of the item. Thisid
should be unique across all items. - If
items
is updated (e.g., items are added, removed, or reordered), React Native uses the keys to efficiently update the UI by reordering, adding, or deleting theText
components as needed instead of re-rendering the entire list.
Why Not Use Index as Key?
Using the index of the item in the array as a key is a common practice, but it can lead to performance issues and bugs in certain scenarios, such as:
- If the order of items changes, the component state can become mixed up, as React reuses the component based on its key.
- If items are added or removed from the beginning or middle of the list, indices change, causing unnecessary re-renders or incorrect element recycling.
Therefore, it's best to use unique and stable identifiers from your data as keys whenever possible. This approach reduces the chances of rendering bugs and improves the efficiency of updates in React Native applications.