Managing Focus and App States in React Native
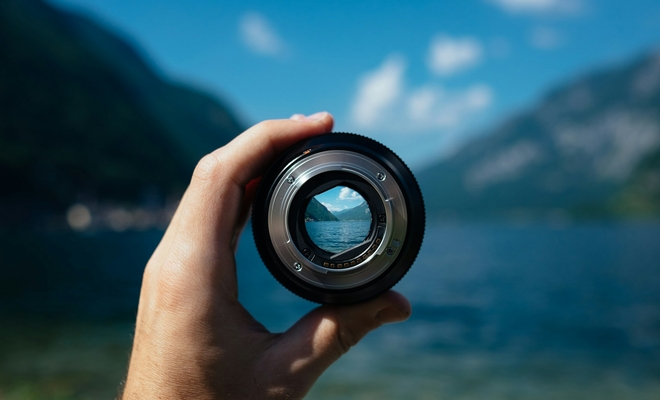
Managing focus and handling background and foreground states in a React Native application are crucial for creating smooth and user-friendly experiences. Properly handling these aspects ensures that your app maintains data integrity, conserves resources, and provides seamless interactions.
Managing Focus
In React Native, managing focus typically revolves around text inputs and similar form elements. React Native provides methods on text input components to programmatically manage focus, blur, and other interactions.
Example Usage:
import React, { useRef } from 'react';
import { TextInput, View, Button } from 'react-native';
const FocusExample = () => {
const inputRef = useRef(null);
const focusOnInput = () => {
inputRef.current.focus();
};
const blurInput = () => {
inputRef.current.blur();
};
return (
<View>
<TextInput ref={inputRef} />
<Button title="Focus" onPress={focusOnInput} />
<Button title="Blur" onPress={blurInput} />
</View>
);
};
In this example, focus()
and blur()
are methods provided by the TextInput
component that can be called to control focus programmatically.
Managing App Background and Foreground States
Handling app state transitions (background and foreground) is crucial for tasks like saving data, releasing resources, or updating the user interface in response to state changes. React Native provides several lifecycle events and hooks through libraries to manage these states.
Using AppState
AppState
can tell you if the app is in the foreground or background and notify you when the state changes. This is useful for performing actions like pausing/resuming animations or handling other interactive tasks.
Example Usage:
import React, { useEffect, useState } from 'react';
import { AppState, Text } from 'react-native';
const AppStateExample = ()okeStateExample{
const [appState, setAppState] = useState(AppState.currentState);
useEffect(() => {
const subscription = AppState.addEventListener("change", nextAppState => {
setAppState(nextAppState);
});
return () => {
subscription.remove();
};
}, []);
return <Text>Current app state: {appState}</Text>;
};
This component listens for changes in the app state and updates its state accordingly, which could then be used to trigger other effects or actions.
Best Practices
-
Focus Management:
- Ensure Accessibility: When managing focus, especially in forms or navigation, ensure that the focus order makes sense from an accessibility perspective.
- Manage Keyboard Visibility: When focusing on text inputs, handle the keyboard appropriately by using libraries like
KeyboardAvoidingView
to ensure that the UI accommodates the keyboard.
-
AppState Management:
- Conserve Resources: Use background and foreground state changes to conserve resources. For example, disconnect from backends or release system resources when the app goes into the background.
- Save User Data: Always save any user progress or data before going into the background to prevent data loss.
-
Testing: Test focus management and app state handling across different devices and OS versions to ensure consistent behavior.
By effectively managing focus and app state transitions, you can enhance the user experience, increase the efficiency of your app, and ensure smoother interactions in React Native applications.