Offloading Tasks to the Background in React Native
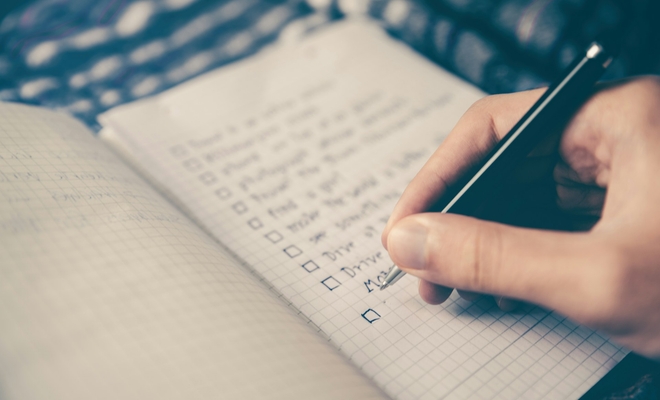
Introduction
In mobile application development, it's crucial to maintain smooth and responsive interfaces to deliver a good user experience. Heavy computations or long-running tasks can cause noticeable lag or even freeze the app if executed on the main thread. Offloading tasks to the background in React Native helps mitigate this issue, ensuring that the UI remains responsive and smooth. This can be achieved by using Web Workers or by offloading tasks to background threads.
Web Workers
Web Workers provide a mechanism to run scripts in background threads, allowing web applications to manage concurrency effectively. By offloading tasks to a Web Worker, JavaScript code can execute in parallel with the main thread, ensuring that the UI remains responsive.
Key Features of Web Workers
- Concurrency: Web Workers introduce parallelism to web applications, allowing tasks to run concurrently.
- Non-blocking: Running tasks in a separate thread ensures the main thread is free for UI rendering.
- Communication: Web Workers communicate with the main thread through message passing.
Limitations of Web Workers
- No DOM Access: Web Workers can't directly interact with the DOM.
- Complex Debugging: Debugging Web Workers can be challenging due to their execution in separate threads.
Example Usage of Web Workers
// Creating a new worker
const myWorker = new Worker('worker.js');
// Sending a message to the worker
myWorker.postMessage('Hello');
// Receiving a message from the worker
myWorker.onmessage = function(e) {
console.log('Message received from worker:', e.data);
};
// Worker code in 'worker.js'
self.onmessage = function(e) {
console.log('Message received from main script');
const result = e.data.toUpperCase();
postMessage(result);
};
Offloading to Background Tasks
Offloading tasks to the background is not limited to Web Workers. It involves techniques that offload non-UI tasks to a separate process:
Techniques for Offloading Tasks
- Asynchronous Programming: Leveraging async/await, Promises, and callbacks for non-blocking operations.
- Service Workers: For handling network requests, caching, push notifications, and background sync.
- Web Workers: For computationally expensive tasks.
- Libraries and Frameworks: Tools like Workbox, Redux-Saga, and RxJS for managing side effects and background tasks.
Best Practices for Background Tasks
- Resource Management: Ensure that background tasks are efficiently managed to avoid memory leaks.
- User Experience: Minimize the impact on device resources like battery life and data usage.
- Security: Sanitize data exchanged with Web Workers to prevent script injection attacks.
By thoughtfully offloading tasks to the background, React Native developers can maintain responsive UIs, improve user experience, and handle resource-intensive operations effectively.