Optimizing Performance in React Native for Complex, Image-Heavy, and Large Lists
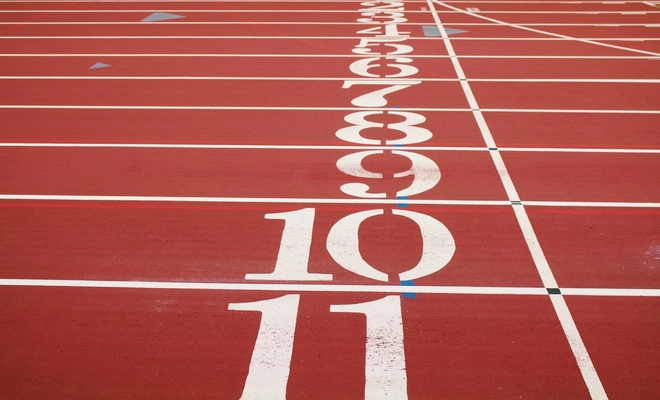
Optimizing Performance in React Native for Complex, Image-Heavy, and Large Lists
Introduction
React Native, an open-source framework created by Facebook, enables developers to build mobile applications using JavaScript while sharing a significant portion of code across iOS and Android platforms. However, one of the most notable challenges faced by developers working with React Native is performance, especially when dealing with complex lists and image-heavy content.
The Problem: Performance Challenges in React Native
When an application contains large lists, such as a feed of posts or a catalog of products, the sheer volume of data can overwhelm the rendering process, resulting in sluggish scrolling and poor user experience. Similarly, handling image-heavy content, such as photo galleries or image backgrounds, can lead to high memory usage and delayed loading times, further degrading performance.
React Native's default components, like ScrollView
and Image
, while functional, are not optimized for handling vast amounts of data or high-resolution images. This often leads to performance bottlenecks, causing lagging and janky interactions.
Efficient List Rendering
FlatList
andSectionList
Components: These components are designed for large data sets, rendering only the items visible on the screen to save memory and processing power.- Props for Performance: Adjust
initialNumToRender
,maxToRenderPerBatch
, andwindowSize
properties to control how many items are rendered initially, in batches, and in the viewable area, respectively.
Image Optimization
- Image Caching and Compression: Implement image caching to store images locally, reducing network requests, and compress images to minimize load time and bandwidth usage.
- Use Appropriate Image Component: Use third-party libraries like
react-native-fast-image
for efficient image rendering and caching. - Resize Images: Properly size images for mobile devices to avoid excessive memory and processing consumption.
Optimize Rendering
- Avoid Unnecessary Rerenders: Utilize
React.memo
,useMemo
, anduseCallback
to prevent needless re-renders. - Optimize Component Keys: Ensure each item in a list has a unique key to aid React Native in managing updates efficiently.
Memory Management
- Manage Memory Usage: Monitor memory usage closely, unloading resources when not needed, and utilize profiling tools to detect memory leaks.
- Use Efficient Data Structures: Choose appropriate data structures for optimal memory and processing efficiency.
Incremental Loading
- Pagination and Infinite Scrolling: Load data incrementally through pagination or infinite scrolling to improve initial load time and reduce memory usage.
Background Processing
- Offload Tasks: Use libraries like
react-native-background-task
orreact-native-background-fetch
to manage data fetching or computations in the background.
Use of Native Modules
- Leverage Native Code: Implement critical parts of your app in Swift (for iOS) or Java/Kotlin (for Android) to utilize platform-specific performance benefits.
Conclusion
By incorporating these strategies, you can significantly improve the performance of React Native applications, particularly when dealing with complex, image-heavy, or large lists. Regularly profiling and testing on real devices is essential to identify and fix potential performance bottlenecks, ultimately providing a smoother experience for users.