Building an iOS Bridge in React Native
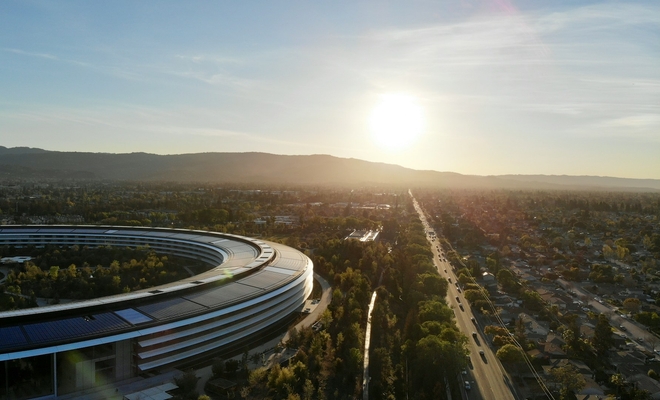
Introduction
React Native is a robust framework, but sometimes you'll need features that aren't supported natively. To access such features, you can write a bridge to connect JavaScript code with native iOS code. This guide walks you through building an iOS bridge using Objective-C.
Prerequisites
- Xcode: Make sure Xcode is installed for iOS development.
- React Native CLI: Install the React Native CLI.
- Objective-C or Swift: Familiarity with Objective-C or Swift for iOS development.
Step-by-Step Guide
1. Create a New React Native Project
If you don't have a project yet, create one:
npx react-native init MyProject
cd MyProject
2. Add a Native Module
We'll focus on Objective-C to write a bridge for iOS.
-
Create the Native Module Header File
In the
ios
directory, add a new.h
header file, for example,MyModule.h
.#import <React/RCTBridgeModule.h> @interface MyModule : NSObject <RCTBridgeModule> @end
The
RCTBridgeModule
protocol from theReact
library defines the interface for the module. -
Implement the Native Module
Add a
.m
implementation file in the same directory. For example,MyModule.m
.#import "MyModule.h" #import <React/RCTLog.h> @implementation MyModule // Register the module with React Native RCT_EXPORT_MODULE(); // Method that logs a message RCT_EXPORT_METHOD(printLog:(NSString *)message) { RCTLogInfo(@"%@", message); } @end
- The
RCT_EXPORT_MODULE()
macro registers the module. - The
RCT_EXPORT_METHOD
macro exposes theprintLog
method, which accepts a string and logs it.
- The
3. Expose the Native Module to JavaScript
Create a JavaScript wrapper to access the native module. Add a file named MyModule.js
in your JavaScript source folder:
import { NativeModules } from 'react-native';
const { MyModule } = NativeModules;
export default {
printLog(message) {
MyModule.printLog(message);
},
};
4. Use the Native Module in React Native
Use the printLog
method in your React Native code:
import React from 'react';
import { View, Button } from 'react-native';
import MyModule from './MyModule';
const App = () => {
return (
<View>
<Button
title="Print Log"
onPress={() => {
MyModule.printLog('Hello from Native Module!');
}}
/>
</View>
);
};
export default App;
Conclusion
Writing an iOS bridge allows you to extend the capabilities of your React Native app by leveraging native iOS APIs and features. This flexibility lets you implement features not natively available in React Native, providing more control over your app's behavior and features.