Understanding the Virtual DOM in React
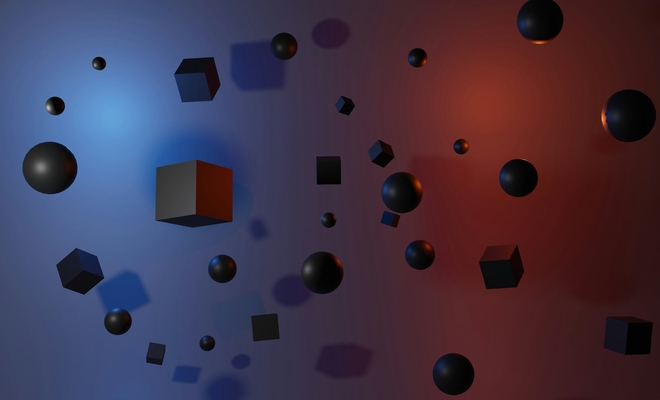
Understanding the Virtual DOM in React
The Virtual DOM (Document Object Model) is a foundational concept in React that enhances the efficiency of updates to the user interface (UI). It's a lightweight abstraction of the "real" DOM, which is a structured representation of the UI elements on a web page. React uses this Virtual DOM to optimize rendering and make UI updates faster and more scalable.
How the Virtual DOM Works
-
Representation:
- The Virtual DOM is essentially a JavaScript object that represents the DOM tree. It includes the elements, their attributes, and their content, but it doesn't have the power to directly change what's on the screen.
-
Reconciliation:
- When a React component's state or props change, the component re-renders, meaning React creates a new Virtual DOM tree representing the updated UI.
- React then compares this new Virtual DOM tree with the previous one in a process called "diffing". It calculates the most efficient way to update the real DOM to match the new Virtual DOM.
-
Batching Updates:
- Instead of updating the real DOM as soon as changes occur in a component, React "batches" these changes to optimize performance. This means that multiple changes can be executed together in a single DOM update cycle, minimizing direct manipulation of the DOM, which is costly in terms of performance.
-
Efficient Updates:
- After computing the differences between the old and the new Virtual DOM, React updates the real DOM nodes only where changes have occurred. This selective updating prevents the costly operation of re-rendering the entire UI, thus improving the performance of complex applications.
Benefits of the Virtual DOM
- Performance: By minimizing direct interactions with the real DOM and batching updates, React can work much faster than if it directly manipulated the DOM on every update.
- Simplicity: Developers can code as if the entire document is rendered on each change, while React takes care of optimizing updates and redrawing only what’s necessary.
- Maintainability: The decoupling of the actual rendering process (manipulating the real DOM) from the developer's components allows for clearer and more maintainable code.
Limitations
- Overhead: The process of diffing and reconciling requires some computational resources, which means there's an inherent overhead compared to directly manipulating the DOM in simple, static web pages.
- Not a Silver Bullet: For extremely high-frequency updates (like animations), using Virtual DOM might not always be the best approach, and direct DOM manipulation or using Canvas/WebGL might be preferable.
Conceptualizing the Virtual DOM
The Virtual DOM in React isn't exposed directly as a simple JSON object in the source code; it's an abstraction managed internally by React's reconciliation and rendering mechanisms. However, for educational purposes, we can conceptualize what a simplified version of the Virtual DOM might look like as a JavaScript object.
Here’s an example that illustrates how the Virtual DOM might represent a simple HTML structure like this:
<div id="app">
<h1>Hello, World!</h1>
<p>This is a paragraph.</p>
</div>
The corresponding Virtual DOM representation could look something like this in a JavaScript object format:
{
type: 'div',
props: {
id: 'app',
children: [
{
type: 'h1',
props: {
children: "Hello, World!"
}
},
{
type: 'p',
props: {
children: "This is a paragraph."
}
}
]
}
}
Breakdown:
- type: Specifies the type of the DOM node (e.g., 'div', 'h1', 'p').
- props: An object containing properties for this node. This includes:
- id, className, style, etc., which are all standard HTML attributes.
- children: Can be a single element, an array of elements, or text content. This represents the nested structure of the DOM.
This structure is a simplified view of how React might internally manage the representation of a component tree in memory. Each element in the tree is represented as an object with properties and children, making it easy for React to traverse and compare nodes during the diffing process.
Actual React Element
React elements, which are the building blocks of the Virtual DOM, are created using React.createElement
or JSX syntax. Here is what an actual React element might look like in code if you were to console log it:
const element = React.createElement('div', {id: 'app'},
React.createElement('h1', null, 'Hello, World!'),
React.createElement('p', null, 'This is a paragraph.')
);
console.log(element);
When rendered using JSX, which is syntactic sugar over React.createElement
, it looks more familiar to the typical HTML:
const element = (
<div id="app">
<h1>Hello, World!</h1>
<p>This is a paragraph.</p>
</div>
);
These snippets create a React element that internally corresponds to a structure like the JSON object shown above, but it's important to remember that React elements are more complex objects including various methods and properties managed by React.