Understanding Side Effects with `useEffect` and `useLayoutEffect` in React
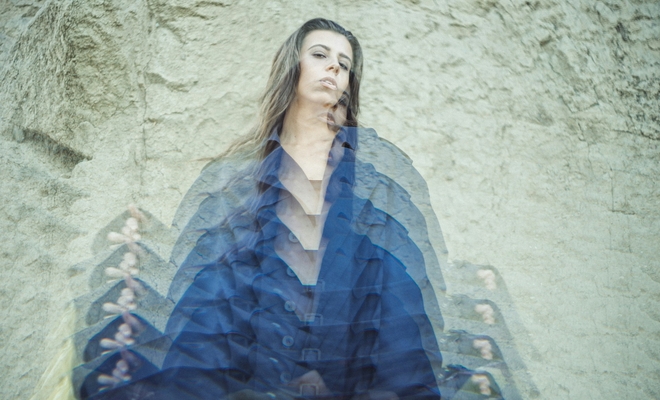
In React, side effects refer to any operation or behavior that affects something outside of the component itself, such as fetching data, directly manipulating the DOM, or setting up subscriptions. These actions occur after the rendering of the component, and managing them efficiently is crucial for optimal performance and a smooth user experience.
Both useEffect
and useLayoutEffect
are hooks designed to manage side effects in functional components. Although they serve similar purposes, they differ in the timing of their execution, which makes them suitable for different use cases.
Timing of Execution
useEffect
:
- Executes after paint. This means the DOM has been updated and the browser has painted the changes before
useEffect
runs, but before the changes become visible to the user. - This non-blocking behavior makes
useEffect
suitable for most effects, particularly those that don't require immediate synchronization with DOM updates, such as data fetching or setting up subscriptions.
useLayoutEffect
:
- Executes synchronously after all DOM mutations but before the browser has had a chance to paint the updated screen. This ensures that any updates scheduled within
useLayoutEffect
are completed in the same visual update cycle that caused them. - Due to its synchronous nature,
useLayoutEffect
is essential for effects that need to measure or mutate the DOM, ensuring visual synchronization without flickering or noticeable delays.
Use Cases
When to use useEffect
:
- For data fetching, setting up a subscription, or other non-UI work that doesn't need to block DOM paint.
- When your effect doesn't interact with the DOM or require measurements to happen immediately.
- For effects that can occur after the screen update, like sending analytics data or logging.
When to use useLayoutEffect
:
- For directly interacting with the DOM or reading layout information from the DOM before the browser paints.
- When you need to make visual updates in response to a change and want to avoid flickering or visual inconsistency, such as adjusting scroll position after an update.
Example Scenarios
useEffect
Example:
useEffect(() => {
// Fetch data from an API and set it to state
fetchData().then(data => setState(data));
}, []); // The empty dependencies array ensures this runs only once on mount
useLayoutEffect
Example:
useLayoutEffect(() => {
// Measure the height of an element immediately after it has been updated
const height = ref.current.clientHeight;
console.log(height); // Do something with the height, like adjusting sibling components or triggering animations
}, [dependencies]); // This runs right after DOM updates but before the paint
Performance Considerations
-
Performance Impacts: Since
useLayoutEffect
runs synchronously after DOM changes but before paint, it can block visual updates if the operations inside it are heavy, leading to decreased performance. Thus, it should be used sparingly and only when necessary to avoid causing frame drops or sluggish UI updates. -
Default Choice: By default, use
useEffect
unless there is a specific reason related to layout and visual updates that requiresuseLayoutEffect
. This approach helps keep your component performance optimized.
By understanding the differences between useEffect
and useLayoutEffect
and choosing the appropriate hook based on the effect's interaction with the DOM and its timing, you can ensure smoother visual updates and better overall performance in your React applications.