Styling Components in React and React Native: Methods, Best Practices, and Tips
April 18, 20244 min read
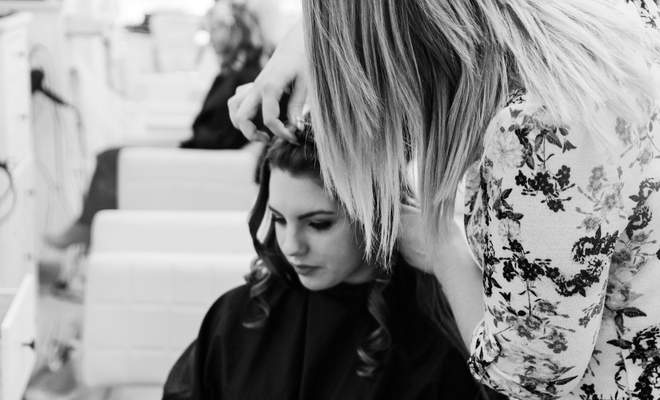
Styling Components in React and React Native: Methods, Best Practices, and Tips
Styling components in React and React Native differs in their approach due to the environments they target—web for React and mobile platforms for React Native. Let's explore the various methods for styling components in each ecosystem and highlight the best practices for each.
React Styling Methods
-
CSS Modules
- Best for: Scalability and avoiding style conflicts.
- Practice: CSS Modules allow CSS to be imported and used in JavaScript, with class names scoped locally by default to prevent global namespace pollution.
-
Styled Components (or other CSS-in-JS libraries)
- Best for: Component encapsulation and dynamic styling based on props.
- Practice: Styled Components enable writing CSS directly within JavaScript code, creating scoped styles that are tightly coupled with components.
-
Emotion
- Best for: High performance and flexibility.
- Practice: Emotion is a CSS-in-JS library that supports both styled and CSS prop approaches, offering flexibility and optimization options.
-
Tailwind CSS
- Best for: Rapid UI development and utility-first design.
- Practice: Tailwind is a utility-first CSS framework used to build custom designs directly in JSX with its array of utility classes.
-
Inline Styles
- Best for: Simple applications and quick prototyping.
- Practice: Passing a style object directly to the
style
prop is quick but limited in features like pseudo-classes or media queries.
-
Global CSS
- Best for: Broad styling and resets.
- Practice: Global CSS files are useful for defining resets or base typography that applies across the entire application.
-
Sass/LESS
- Best for: Complex applications needing CSS preprocessing.
- Practice: Pre-processors like Sass or LESS integrate into React projects to utilize features like variables, mixins, and nested syntax.
Best Practices for React Styling
- Consistency: Stick to one styling method across your project to keep the codebase maintainable.
- Component Scope: Use local or scoped styling methods to reduce side effects and enhance component reusability.
- Performance Considerations: Consider the performance implications, especially with CSS-in-JS libraries in large-scale applications.
- Accessibility: Ensure your styling approach maintains accessibility standards.
React Native Styling Methods
-
StyleSheet API
- Best for: Performance and common use.
- Practice: React Native's
StyleSheet
API is the most common way to style components, providing optimized performance.
-
Inline Styles
- Best for: Simple applications and quick prototyping.
- Practice: Inline styles in React Native are similar to inline styles in web-based React but should be used sparingly due to performance considerations.
-
Styled Components
- Best for: Component encapsulation and dynamic styling.
- Practice: Styled Components can be used in React Native to write CSS-in-JS, beneficial for conditional styling based on props.
-
Emotion or other CSS-in-JS libraries
- Best for: Extended styling capabilities with JavaScript.
- Practice: Similar to Styled Components, other CSS-in-JS libraries like Emotion can be adapted for React Native.
-
Tailwind CSS (via Twin or other libraries)
- Best for: Utility-first design and rapid development.
- Practice: Libraries like Twin enable Tailwind CSS for React Native, allowing for utility-based UI development.
Best Practices for React Native Styling
- Optimize Performance: Prefer
StyleSheet.create
to define styles outside of the render method. - Responsive Design: Use Flexbox properties to build layouts that work across different screen sizes and orientations.
- Platform-Specific Styles: Utilize the
Platform
module to apply styles conditionally based on the operating system. - Scalability: Consider structured approaches like CSS-in-JS if dynamic theming and props-based styling become prevalent.
- Accessibility: Ensure sufficient color contrast, text size, and interactive element sizing.
Both ecosystems offer diverse methods and tools for styling components. The choice of the best method depends on your project's requirements, team expertise, and the need for performance optimization.