Understanding Webpack: The Modern JavaScript Module Bundler
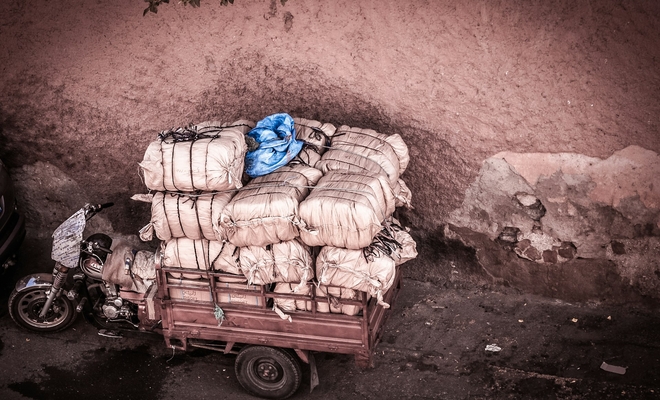
Understanding Webpack: The Modern JavaScript Module Bundler
Webpack is a popular open-source JavaScript module bundler. It’s primarily used for bundling JavaScript files, but it can also transform, bundle, or package any resource or asset. Webpack helps developers manage dependencies and optimize assets, making it a powerful tool for modern front-end development.
Key Features of Webpack
-
Module Bundling: Webpack analyzes the dependency graph of a project and bundles the code into one or more files. This bundling process helps reduce the number of HTTP requests needed to load an application.
-
Code Splitting: Webpack can split the code into multiple bundles, which are then loaded on-demand or in parallel. This helps to optimize performance by only loading the necessary parts of the application.
-
Loaders: Loaders allow webpack to preprocess files as they are imported, transforming non-JavaScript files (like CSS, images, and JSON) into JavaScript modules. This enables webpack to handle many different types of assets.
-
Plugins: Webpack plugins extend webpack's functionality to handle a wide range of tasks, like minification, file generation, optimization, and hot module replacement.
-
Tree Shaking: Webpack’s tree shaking feature allows it to remove dead code from bundles, which helps reduce the final size of the output.
-
Development Server: The webpack development server provides live reloading, allowing developers to see changes to their code immediately without refreshing the browser.
-
Asset Management: It can handle static assets like images, fonts, and stylesheets, making it easier to include these assets in the JavaScript bundle.
How Webpack Works
-
Entry Point: Webpack starts the bundling process from an entry point, which is typically the main JavaScript file of the application.
-
Dependency Graph: From the entry point, webpack recursively analyzes the dependency graph to identify all files that are directly or indirectly referenced.
-
Modules: Webpack treats each file as a module. It processes each module through loaders and plugins to transform the code.
-
Output: The processed modules are bundled into one or more output files, which are served to the browser or another environment.
Basic Webpack Configuration
A basic webpack configuration file (webpack.config.js
) typically contains:
-
Entry: Specifies the entry point(s) for the application.
module.exports = { entry: './src/index.js', // ... };
-
Output: Defines where the bundled files will be output.
module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist'), }, };
-
Loaders: Set up loaders to process different types of files.
module.exports = { module: { rules: [ { test: /\.css$/, use: ['style-loader', 'css-loader'], }, ], }, };
-
Plugins: Include plugins to enhance webpack’s capabilities.
const HtmlWebpackPlugin = require('html-webpack-plugin'); module.exports = { plugins: [ new HtmlWebpackPlugin({ template: './src/index.html', }), ], };
Common Plugins and Loaders
- babel-loader: Transpiles modern JavaScript to older versions for compatibility.
- css-loader: Resolves CSS imports into JavaScript.
- file-loader: Moves files to the output directory and provides the correct URLs.
- html-webpack-plugin: Generates an HTML file that automatically includes the JavaScript bundle.
Benefits of Webpack
- Optimized Asset Management: Handles assets like CSS, images, and fonts efficiently, reducing the size of the final bundle.
- Modular Codebase: Encourages a modular structure by treating each file as a module.
- Performance Optimization: Code splitting and tree shaking optimize performance by loading only necessary code.
- Flexibility: Supports a wide range of loaders and plugins for handling different file types and performing various optimizations.
Conclusion
Webpack is a versatile and powerful tool that helps developers manage dependencies, optimize assets, and streamline the development process. Its flexibility and extensive plugin ecosystem have made it a key component in the modern web development stack.